VBA Attributes
VBA code modules contain attributes which can be used to set certain properties of the module and its members. Attributes cannot be seen in the Visual Basic Editor. To see the attributes of a code module, export the module and open the module in a text editor. Attributes can be added or changed and then the file can be imported back into the VBA project so they can take effect. Attributes can be used to set the name of a module or class, add descriptions to code resources, give classes a default global instance, set the default member of a class, and set a class's enumerator for use with For Each loops. Certain attributes can be seen in the exported file but do not have any effect in VBA. In general, it is not necessary and not recommended to set attributes.
Attribute | Description |
---|---|
VB_Name | Used to set the name of the module or class. |
VB_[Var]Description | Used to set description text for modules, classes, procedures, and variables. |
VB_PredeclaredId | Setting to True creates a default global instance of the class when the application starts. |
VB_Exposed | Setting to True makes a class type available outside of the VBA project. Objects of the class type can exist outside the VBA project but cannot be instantiated outside the VBA project. Instances created from an exposed class must be passed from a factory function defined within the VBA project. |
VB_[Var]UserMemId | Setting to 0 makes the member the default member. Setting to -4 makes the member the enumerator of a class to be used with For Each loops. |
Attribute | Description |
---|---|
VB_GlobalNameSpace | *No effect in VBA. Makes the class's scope global so the name of the class does not need to be used to reference class members. |
VB_Creatable | *No effect in VBA. Setting to True allows a class to be instantiated outside of the VBA project in which the class is defined. |
VB_MemberFlags | *No effect in VBA. Setting to "40" makes member hidden. |
VB_Name
The VB_Name attribute can be used to set the name of a module or class. This attribute can also be set in the Properties window in the Visual Basic Editor.
VERSION 1.0 CLASS
BEGIN
MultiUse = -1 'True
END
Attribute VB_Name = "clsExample"
Attribute VB_GlobalNameSpace = False
Attribute VB_Creatable = False
Attribute VB_PredeclaredId = False
Attribute VB_Exposed = False
Option Explicit
Public Message As String
Public Sub Example()
Debug.Print Message
End Sub
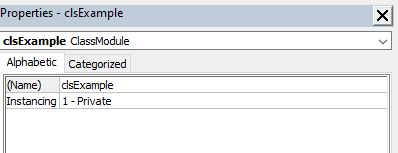
VB_[Var]Description
The VB_[Var]Description attribute can be set description text for modules, classes, procedures, and variables. When declaring the attribute for a module use the form "VB_Description". When declaring the attribute for a procedure use the form "ProcedureName.VB_Description" inside the procedure. When declaring the attribute for a variable use the form "VariableName.VB_VarDescription".
VERSION 1.0 CLASS
BEGIN
MultiUse = -1 'True
END
Attribute VB_Name = "clsExample"
Attribute VB_Description = "This is a description of a class"
Attribute VB_GlobalNameSpace = False
Attribute VB_Creatable = False
Attribute VB_PredeclaredId = False
Attribute VB_Exposed = False
Option Explicit
Public Message As String
Attribute Message.VB_VarDescription = "This is a description of a member variable"
Public Sub Example()
Attribute Example.VB_Description = "This is a description of a procedure"
Debug.Print Message
End Sub
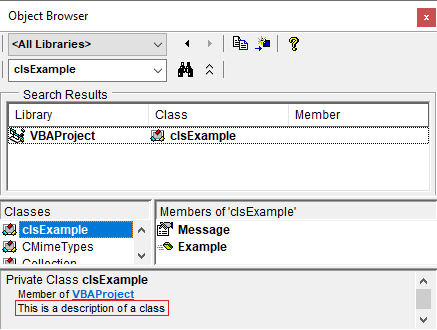
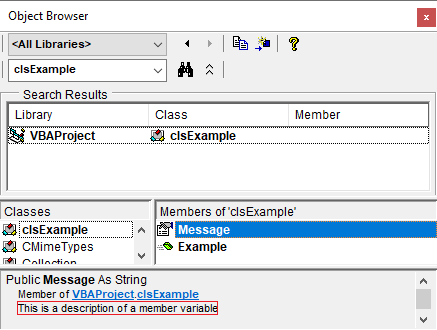
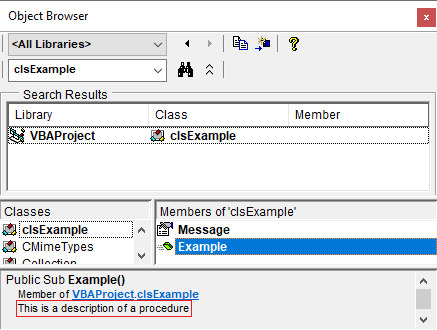
VB_PredeclaredId
The VB_PredecalredId attribute can be used to create a default global instance of a class. Setting this attribute to True will cause a global instance to be created when the application starts.
Option Explicit
Public Sub Example()
Dim TheSum As Double
TheSum = MyMathFunctions.Add2Numbers(3.5, 3.5)
Debug.Print TheSum
End Sub
VERSION 1.0 CLASS
BEGIN
MultiUse = -1 'True
END
Attribute VB_Name = "MyMathFunctions"
Attribute VB_GlobalNameSpace = False
Attribute VB_Creatable = False
Attribute VB_PredeclaredId = True
Attribute VB_Exposed = False
Option Explicit
Public Function Add2Numbers(Num1 As Double, Num2 As Double) As Double
Add2Numbers = Num1 + Num2
End Function
VB_Exposed
The VB_Exposed attribute is used to specify the accessibility of a class. When set to True this attribute makes a class type visible to outside VBA projects. This has the same effect as setting the Instancing property of a class to PublicNotCreatable. By default this property is set to False which makes the Instancing property Private. PublicNotCreateable classes allow variables of the class type to be declared outside of the VBA project in which they are defined but objects of the class type cannot be instantiated outside the VBA project in which they are defined. A public factory function within the VBA project can be used to instantiate the class instance which can be called from outside the VBA project.
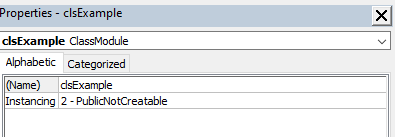
VB_[Var]UserMemId
The VB_[Var]UserMemId attribute can be used to set a default member of a class and to set an enumerator for a class to be used with For Each loops.
Default Member
To make a member the default member of a class use the form "MemberName.[Var]UserMemId" and set the attribute to 0. Member variables or procedures can be the default member. It is most common to have a Property be the default member.
Option Explicit
Public Sub Example()
Dim E As clsExample
Set E = New clsExample
'The Message property is the default member
E = "Hello, World!"
Debug.Print E
End Sub
VERSION 1.0 CLASS
BEGIN
MultiUse = -1 'True
END
Attribute VB_Name = "clsExample"
Attribute VB_Description = "This is a description of a class"
Attribute VB_GlobalNameSpace = False
Attribute VB_Creatable = False
Attribute VB_PredeclaredId = False
Attribute VB_Exposed = False
Option Explicit
Private pMessage As String
Attribute pMessage.VB_VarDescription = "Private Message Variable"
Public Property Get Message() As String
Attribute Message.VB_Description = "This is a description of a member variable"
Attribute Message.VB_UserMemId = 0
Message = pMessage
End Property
Public Property Let Message(RHS As String)
Attribute Message.VB_Description = "This is a description of a member variable"
Attribute Message.VB_UserMemId = 0
pMessage = RHS
End Property
Public Sub Example()
Attribute Example.VB_Description = "This is a description of a procedure"
Debug.Print Message
End Sub
Enumerator
To return an enumerator from a procedure have the procedure return the [_NewEnum] hidden property of a Collection object as an IUknown interface type. Use the form "PropertyName.VB_UserMemId" and set the attribute to -4 to indicate that the property is an enumerator.
Option Explicit
Public Sub Example()
Dim E As clsExample
Set E = New clsExample
Set E.Coll = New Collection
E.Coll.Add 1
E.Coll.Add 2
E.Coll.Add 3
Dim i As Variant
For Each i In E
Debug.Print i
Next i
End Sub
VERSION 1.0 CLASS
BEGIN
MultiUse = -1 'True
END
Attribute VB_Name = "clsExample"
Attribute VB_Description = "This is a description of a class"
Attribute VB_GlobalNameSpace = False
Attribute VB_Creatable = False
Attribute VB_PredeclaredId = False
Attribute VB_Exposed = False
Option Explicit
Public Coll As Collection
Attribute Coll.VB_VarDescription = "This is a description of a member variable"
Public Property Get NewEnum() As IUnknown
Attribute NewEnum.VB_UserMemId = -4
Set NewEnum = Coll.[_NewEnum]
End Property
Public Sub Example()
Attribute Example.VB_Description = "This is a description of a procedure"
Debug.Print Message
End Sub